Content Enricher
Camel supports the Content Enricher from the EIP patterns.
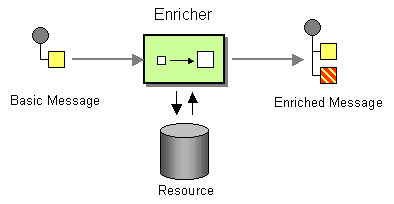
In Camel the Content Enricher can be done in several ways:
-
Using Enrich EIP
-
Using a Message Translator
-
Using a Processor with the enrichment programmed in Java
-
Using a Bean EIP with the enrichment programmed in Java
The most natural Camel approach is using Enrich EIP.
Content enrichment using a Message Translator
You can consume a message from one destination, transform it with something like Velocity, XQuery, or AtlasMap and then send it on to another destination.
from("activemq:My.Queue")
.to("velocity:com/acme/MyResponse.vm")
.to("activemq:Another.Queue");
And in XML
<route>
<from uri="activemq:My.Queue"/>
<to uri="velocity:com/acme/MyResponse.vm"/>
<to uri="activemq:Another.Queue"/>
</route>
You can also enrich the message in Java DSL directly (using fluent builder) as an Expression. In the example below the message is enriched by appending ` World!` to the message body:
from("direct:start")
.setBody(body().append(" World!"))
.to("mock:result");
The fluent builder is not available in XML or YAML DSL, instead you can use Simple language:
<route>
<from uri="direct:start"/>
<setBody>
<simple>${body} World!</simple>
</setBody>
<to uri="mock:result"/>
</route>
Content enrichment using a Processor
In this example we add our own Processor using explicit Java to enrich the message:
from("direct:start")
.process(new Processor() {
public void process(Exchange exchange) {
Message msg = exchange.getMessage();
msg.setBody(msg.getBody(String.class) + " World!");
}
})
.to("mock:result");
Content enrichment using a Bean EIP
we can use Bean EIP to use any Java method on any bean to act as content enricher:
from("activemq:My.Queue")
.bean("myBeanName", "doTransform")
.to("activemq:Another.Queue");
And in XML DSL:
<route>
<from uri="activemq:Input"/>
<bean ref="myBeanName" method="doTransform"/>
<to uri="activemq:Output"/>
</route>
Content enrichment using Enrich EIP
Camel comes with two kinds of Content Enricher EIPs:
-
Enrich EIP - This is the most common content enricher that uses a
Producer
to obtain the data. It is usually used for Request Reply messaging, for instance to invoke an external web service. -
Poll Enrich EIP - Uses a Polling Consumer to obtain the additional data. It is usually used for Event Message messaging, for instance to read a file or download a FTP file.
For more details see Enrich EIP and Poll Enrich EIP.